Friday, December 26, 2008
Should I make a new wheel?
Tuesday, December 16, 2008
clean_data and cleaned_data
>>>python manage.py shell
>>>from pageage1 import MyForm
>>>form=MyForm({'username':'Eric','email':'test@test.com'})
>>>form.is_valid()
True
We could access the form's data by attribute form.data, If it's validated we can also access the valid data by attribute form.clean_data,but when you upgrade to Django 1.0,this attribute will be no long in use.Instead of it,you should use the attribute cleaned_data:
...
>>>form.clean_data # Django 0.96
>>>form.cleaned_data # Django 1.0
Monday, December 15, 2008
errors and has_errors
<div style="margin-left: 40px;"> <!DOCTYPE html PUBLIC "-//W3C//DTD HTML 4.01 Transitional//EN" "http://www.w3.org/TR/html4/loose.dtd">
<html>
<head>
<meta equiv="Content-Type" content="text/html; charset=ISO-8859-1">
<title>Django Bookmarks - User Login</title>
</head>
<body>
<h1>User Login</h1>
{%if form.has_errors%}
<p>Your username and password didn't match.
Please try again.</p>
{%endif%}
<form method="post" action=".">
<p><label for="id_username">Username:</label>
{{ form.username }}</p>
<p><label for="id_password">Password:</label>
{{ form.password }}</p>
<input type="hidden" name="next" value="/">
<input type="submit" value="login">
</form>
</body>
</html>
In version 0.95,it's work fine.but if you run it under the version 1.0,you can't see the error message when you login-failing.The question is in Django 1.0,the attribute of form object is errors instead of has_error.so change the attribute it'll work well.
Thursday, November 27, 2008
How to write something in PDF by python
#first we import necessary module.
from reportlab.pdfgen import canvas
#now,open the file which we want to covert to
f=open("c:/log.txt","rb")
#read each line in the file.
lines=f.readlines()
#create a canvas objects,it can write the string
#in the pdf
c=canvas.Canvas("c:/h.pdf")
#maybe the file we read was very big so we must
#consider that display them in multiple page
j=700 #for example it's height is 700 point
for row in lines:
#firstly, we do an encoding cover.
uniline=unicode(row,'latin-1')
if j==0:
j=700
#showPage method means,we want to write in next page
c.showPage()
else:
j=j-10
#drawString method will draw the text information in PDF
c.drawString(100, j, uniline.strip())
#don't forget close the file
f.close()
#don't forget save the canvas,otherwise,you can't see anything
#in the .pdf file
c.save()
It's simple that is,right?
Wednesday, November 26, 2008
The order of the Python's class inheritance
class A:
def method1(self):
print "this is the method1 of class A"
class B:
def method1(self):
print "this is the method2 of class B"
class C(A,B): #class C inherit from A and B
pass
c=C()
c.method1()#Now the question is which method will be call,A or B
#The result is:
this is the method1 of class A
That means,the inheritance rest with which super class in the starting location,in this example,class A in the zero position,so the method1 in class A will be call instead of class B
Tuesday, November 25, 2008
A better way to create your own python path
Sunday, November 23, 2008
Function argument-matching forms In Python
Syntax | Location | Interpretation |
---|---|---|
func(value) | Caller | Normal argument: matched by position |
func(name=value) | Caller | Keyword argument: matched by name |
def func(name) | Function | Normal argument: matches any by position or name |
def func(name=value) | Function | Default argument value, if not passed in the call |
def func(*name) | Function | Matches remaining positional args (in a tuple) |
def func(**name) | Function | Matches remaining keyword args (in a dictionary |
Python's object reference
list1=[1,2,3]
str1="hello"
def f2(x,y):
list1.append(4)
y="world"
f2(list1,str1)
list1 #the value is [1,2,3,4],it's changed
str1 # the value is "hello",str1 is not changed
Because Python's argument is the object reference,so if you stuff a mutable object,its value will be change in place.sometime if you want to avoid your outside variable changed by function,you can pass a copy of mutable object to the function:
list1=[1,2,3]
str1="hello"
def f2(x,y):
list1.append(4)
y="world"
f2(list1[:],str1) #Now,we assign a list1[:],it's the copy of list1
list1 #the value is [1,2,3],it's not changed
str1 # the value is "hello",str1 is not changed
Or we can use tuple keyword,convert an array object to a tuple object:
list1=[1,2,3]
str1="hello"
def f2(x,y):
list1.append(4)
y="world"
f2(tuple(list1),str1) #Now,we assign a tuple,it's convert from list1
list1 #the value is [1,2,3],it's not changed
str1 # the value is "hello",str1 is not changed
The global statement
class Test{but if you think you can change a module level variable in Python, you are totally wrong.please see following:
private String x=null;//declare a new variable:x
public change_variable(){
x="hello" //change the outside variable inside the method
}
}
#python code
x=99 #this is a global variable
#define a function
def func():
x=100
func() #call this function
print x # what's happened? x=99 or x=100
#the truth is x=99
Why is that,because when you use a same variable name in the function,Python will create a new local variable instead of use the global variable,so the global x is not changed.Then,if you really want to change the global variable,you must use the global keyword:
#python code
x=99 #this is a global variable
#define a function
def func():
global x #now it seems as we declare that we'll use the global x
x=100
func() #call this function
print x # what's happened? x=99 or x=100
#now x=100
Thursday, November 20, 2008
How to find you win32ui.pyd
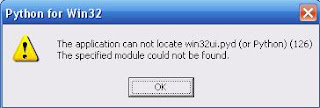
Did you ever have this problem,when you install the Activity Python and run Python win,you will got an exception:'The application cannot locate win32ui.pyd ...'.
To solve it,we just need download and install another python library,pywin32.to download it from https://sourceforge.net/projects/pywin32/ and setup it.
Wednesday, November 19, 2008
How to use zip and map function
#python code:
>>> l1=['x','y','z']
>>> l2=[1,2,3]
>>> zip(l1,l2)
[('x', 1), ('y', 2), ('z', 3)]
>>>
Now,you can loop in this new zip model,and have the benefit from it,for example,you want to loop in these two list at the same time:
#python code:
>>> for (a,b) in zip(l1,l2):
print a,b
x 1
y 2
z 3
>>>
With this benefit,we can also create a dictionary object,for example:
#python code:
>>>d={}
>>> for (k,v) in zip(l1,l2):
d[k]=v
print d
{'x':1,'y':2,'z':3}
>>>
also there is a short way to create a dictionary object,use the build-in function,dict:
#python code:
>>>d={}
>>> d=dict(zip(l1,l2))
{'x':1,'y':2,'z':3}
>>>
Now,how about map function,map function is similar to zip,but for zip if your two list object has different number of items,then the zip function will truncate the larger one.for example:
#python code:
>>>l1=['x','y']
>>>l2=[1,2,3]
>>>zip(l1,l2)
>>>[('x',1),('y',2)]
but if you use the map function,you can stuff another parameter,to tell the function how to display that can't match items:
#python code:
>>>l1=['x','y']
>>>l2=[1,2,3]
>>>map(l1,l2)
>>>[('x',1),('y',2),(None,3)]
Print to a file,a simple log system
Here is the example code:
#python code
>>log=open('c:/log.txt','a') #open a file to log the message
>>print>>log,"This is log information" #then we put the log information to a file
>>log.close() # don't forget close the file
Tuesday, November 18, 2008
Comparisons,Equality in Python
For instance, a comparison of list objects compares all their components automatically:
>>> L1 = [1, ('a', 3)] # Same value, unique objects
>>> L2 = [1, ('a', 3)]
>>> L1 == L2, L1 is L2 # Equivalent? Same object?
(1, 0)
Here, L1 and L2 are assigned lists that are equivalent, but distinct objects. Because of the nature of Python references (studied in Chapter 4), there are two ways to test for equality:
-
The == operator tests value equivalence. Python performs an equivalence test, comparing all nested objects recursively
-
The is operator tests object identity. Python tests whether the two are really the same object (i.e., live at the same address in memory).
In the example, L1 and L2 pass the == test (they have equivalent values because all their components are equivalent), but fail the is check (they are two different objects, and hence two different pieces of memory). Notice what happens for short strings:
>>> S1 = 'spam'
>>> S2 = 'spam'
>>> S1 == S2, S1 is S2
(1, 1)
Here, we should have two distinct objects that happen to have the same value: == should be true, and is should be false. Because Python internally caches and reuses short strings as an optimization, there really is just a single string, 'spam', in memory, shared by S1 and S2; hence, the is identity test reports a true result. To trigger the normal behavior, we need to use longer strings that fall outside the cache mechanism:
>>> S1 = 'a longer string'
>>> S2 = 'a longer string'
>>> S1 == S2, S1 is S2
(1, 0)
Friday, November 14, 2008
Improve the performance of you string
#Python code
#firstly, we define a new string,it's a poem ,a big string
bigstring=""""
Today I saw a butterfly,
as it floated in the air;
Its wings were spread in splendor,
Unaware that I was there.
""""
#secondly, we convert it to a list
biglist=bigstring.split(' ')
biglist[1]='you'
biglist[3]="one"
#convert it back
bigstring=''.join(biglist)
bigstring
Because list is mutable,each time we operate on it,no new object generated.So we have high performance.
Thursday, November 13, 2008
How to read and write the CSV file
#Python code:
import csv # first we need import necessary lib:csv
file=open("c:/Book1.csv") #prepare a csv file for our example
testReader=csv.reader(file,delimiter=' ', quotechar='|')
#now the testReader is an array,so we can iterate it
for row in testReader:
print "|".join(row)
#The result is:
Test1:Test1
Test2:Test2
.....
Mostly the value of a csv file will separate by comma,but sometime may be other App will generate a csv file and separate other quotechar(|,! etc).So csv lib provide a parameter :delimiter and quotechar, so you can custom it by yourself.
Now let's see how to write something into a csv file:
#Python code:
import csv # first we need import necessary lib:csv
file=open("c:/Book1.csv") #prepare a csv file for our example
testWriter = csv.writer(open('Book1.csv', 'w'), delimiter=' ',
quotechar='|', quoting=csv.QUOTE_MINIMAL)
spamWriter.writerow(['Test'] * 5 + ['Wow'])
spamWriter.writerow(['Hello', 'I'm', 'Super Star'])
It's that easy,right?
Wednesday, November 5, 2008
NumPy :a powerful computing library
The fundamental package needed for scientific computing with Python is called NumPy. This package contains:
- a powerful N-dimensional array object
- sophisticated (broadcasting) functions
- basic linear algebra functions
- basic Fourier transforms
- sophisticated random number capabilities
- tools for integrating Fortran code.
- tools for integrating C/C++ code.
Immutability or mutability
>>s="Spam"
"Spam"
In Java you can declare it as:
String s="Spam"
Now,the requirement is we want to access the sub-string of them:
#Python code
>>> s="Spam"
>>> s[0]
'S'
>>> s[0]="z"
Traceback (most recent call last):
File "<stdin>", line 1, in <module>
TypeError: 'str' object does not support item assignment
As you can see,we can access Python string as a sequence by use 'index'but if we want to assign a new value into the item of this string we got an exception,that means the string object in Python is immutable,it's similar to Java and C++,but it's different to Ruby.In Ruby you can assign a new value into the item of a string,and after assignment ,the original string object changed:
#Ruby code
irb(main):001:0> s="Spam"
=> "Spam"
irb(main):002:0> s[0]
=> "S"
irb(main):003:0> s[0]="z"
=> "z"
irb(main):004:0> s
=> "zpam" #the value of s has been changed.
That means the string object is mutable in Ruby.In terms of the core types, numbers, strings, and tuples are immutable; lists and dictionaries are not (they can be changed in-place freely).
Wednesday, October 8, 2008
How to show the full error stack in wxPython
The solution is:
1)run it in command module.
2)switch you method ,app=wx.App() to
app=wx.App(False)
through this way,when there is any error in your application you will get a full error stack,it'll helpful for your developing
How to develope the Adobe FLV program with Python
you can download it from http://aa-project.sourceforge.net/index.html
AAlib is an portable ascii art GFX library.try use it ,maybe you'll find some cool thing on it.
Monday, July 14, 2008
'ManyRelatedManager' object is not iterable
{% if query%}
{%for book in results%}
{%for author in book.authors%}
{%endfor%}
{%endfor%}
{%else%}
{%endif%}
When I run it.I got an error message:
'ManyRelatedManager' object is not iterable.
the solution for this is
change the for loop syntax to:
{%for author in book.authors.all%}
then it'll be done.
Sunday, May 25, 2008
A PDFLib for Python
Thursday, May 22, 2008
site -- Site-specific configuration hook(past from python site)
This module is automatically imported during initialization. The automatic import can be suppressed using the interpreter's -S option.
Importing this module will append site-specific paths to the module search path.
It starts by constructing up to four directories from a head and a tail part. For the head part, it uses sys.prefix
and sys.exec_prefix
; empty heads are skipped. For the tail part, it uses the empty string and then lib/site-packages (on Windows) or lib/python2.5/site-packages and then lib/site-python (on Unix and Macintosh). For each of the distinct head-tail combinations, it sees if it refers to an existing directory, and if so, adds it to sys.path
and also inspects the newly added path for configuration files.
A path configuration file is a file whose name has the form package.pth and exists in one of the four directories mentioned above; its contents are additional items (one per line) to be added to sys.path
. Non-existing items are never added to sys.path
, but no check is made that the item refers to a directory (rather than a file). No item is added to sys.path
more than once. Blank lines and lines beginning with #
are skipped. Lines starting with import
are executed.
For example, suppose sys.prefix
and sys.exec_prefix
are set to /usr/local. The Python 2.5.2 library is then installed in /usr/local/lib/python2.5 (where only the first three characters of sys.version
are used to form the installation path name). Suppose this has a subdirectory /usr/local/lib/python2.5/site-packages with three subsubdirectories, foo, bar and spam, and two path configuration files, foo.pth and bar.pth. Assume foo.pth contains the following:
# foo package configuration
foo
bar
bletch
and bar.pth contains:
# bar package configuration
bar
Then the following directories are added to sys.path
, in this order:
/usr/local/lib/python2.3/site-packages/bar
/usr/local/lib/python2.3/site-packages/foo
Note that bletch is omitted because it doesn't exist; the bar directory precedes the foo directory because bar.pth comes alphabetically before foo.pth; and spam is omitted because it is not mentioned in either path configuration file.
After these path manipulations, an attempt is made to import a module named sitecustomize, which can perform arbitrary site-specific customizations. If this import fails with an ImportError exception, it is silently ignored.
Note that for some non-Unix systems, sys.prefix
and sys.exec_prefix
are empty, and the path manipulations are skipped; however the import of sitecustomize is still attempted.
Tuesday, May 6, 2008
Our wedding day
Thursday, May 1, 2008
How the Decimal is in Python
>>0.1+0.1+0.1-0.3
you will get
>>5.5511151231257827e-017
The reason is cause of your hardware.Then how should we do then we can get a zero?Use Decimal,
>>from decimal import Decimal
>>Decimal(0.1)+Decimal(0.1)+Decimal(0.1)-Decimal(0.3)
>>Decimal(0)
Great,isn't it?
Then consider another condition,if this:
>>1/3
>>0.33333331
but sometime for example we only want to got fist four precision,how to do it?
>>decimal.getcontext().prec=4
>>Decimal(1)/Decimal(3)
Decimal(0.3333)
Wow...fantastic:)
Division in Python
>>1/2
>>0
As you can see,you will get a result 0,but not 0.5. That is Python will return an integer in default.if you want to get a result 0.5,there are two ways you can do it:
first:
>>1.0/2
>>0.5
second:
>>from __future__ imort divisions
>>1/2
>>0.5
if you want return an integer.you should use "//"
>>1//2
>>0
My labor day
Tuesday, April 29, 2008
A powerful Python Graph lib
In recnet days,I want to add some chart in my application,for example,financial report chart.so I shearch many python chart lib,such as pygooglechart,Flashchart,Matplotlib.In the end,I found matplotlib is what I want,it's a python 2D plotting library which produces publication quality figures in a variety of hardcopy formats and interactive environments across platforms. and it's power full,also it's docuemnt and example is very readable and exhaustive,so I decided to use it in my project.
Some screenshot:


Insomnia
Oh,I can't sleep.Why not write some code?
class man:
def insomnia(self):
self.head="ache"
self.lateforwork=True
def sleep(self):
print "go to bed"
while self.tired==True:
print "turn off the light"
print "Zzzzz..."
else:
print "read book"
Monday, April 28, 2008
zip() function,What does that mean and how to use it?
a=[1,2,3]
b=[4,5,6]
c=[]
for x in map(None,a,b):
c.append(x[0]+x[1])
but it's not a good solution,especially that None argument:)
Now,we have another better choose.zip() function.What's it means?
zip() function will return a list of tuples, where each tuple contains the i-th element from each of the argument sequences. The returned list is truncated in length to the length of the shortest argument sequence.
for example:
a=[1,2,3]
b=[4,5,6]
c=[]
for i in zip(a,b):
c.append(x[0]+x[1])
it's better understand,isn't it?
Sunday, April 27, 2008
A beautiful song from JAY
Thursday, April 24, 2008
A windy day
Today is a windy day in Beijing.I prepare to ride my bike,but the wind is too heavy.What a day,I think.At the last I go to work by bus.I think I'll late.but guess what?I got to work in time.Fantastic!
Wednesday, April 23, 2008
JavaScript menu.show menu between different frame.
Today, I need implement a prototype for our customer.In this implement we need a menu.I put the code here:
main.html<html>
<head>
<title>different frame menu from MSDN</title>
<meta http-equiv="Content-Type" content="text/html; charset=gb2312">
</head>
<frameset rows="92,*" cols="*" framespacing="4" frameborder="yes" border="4">
<frame src="top.htm" name="topFrame" scrolling="NO" >
<frame src="bottom.htm" name="mainFrame" id="mainFrame">
</frameset>
<noframes><body>
</body></noframes>
</html>
top.htm
<html>
<head>
<title>MSDN example</title>
<script>
var oPopup = window.createPopup();
function richContext()
{
var lefter2 = event.offsetY+0;
var topper2 = event.offsetX+15;
oPopup.document.body.innerHTML = oContext2.innerHTML;
oPopup.show(0, 15, 210, 84, contextobox);
}
</script>
</head>
<body>
asdasd
<span id="contextobox" style=" cursor:hand; margin-left:5px; margin-right:10px; background:#e4e4e4; width:300; height:40; padding:20px;" onmouseover="richContext(); return false" >Right-click inside this box.</span>
<DIV ID="oContext2" STYLE="display:none">
<DIV STYLE="position:relative; top:0; left:0; border:2px solid black; border-top:2px solid #cccccc; border-left:2px solid #cccccc; background:#666666; height:110px; width:207px;">
<DIV STYLE="position:relative; top:0; left:0; background:#cccccc; border:1px solid black; border-top: 1px solid white; border-left:1px solid white; height:20px; color:black; font-family:verdana; font-weight:bold; padding:2px; padding-left:10px; font-size:8pt; cursor:hand" onmouseover="this.style.background='#ffffff'" onmouseout="this.style.background='#cccccc'" onclick="parent.parent.mainFrame.location.href='http://www.microsoft.com';">
Home</DIV>
<DIV STYLE="position:relative; top:0; left:0; background:#cccccc; border:1px solid black; border-top: 1px solid white; border-left:1px solid white; height:20px; color:black; font-family:verdana; font-weight:bold; padding:2px; padding-left:10px; font-size:8pt; cursor:hand" onmouseover="this.style.background='#ffffff'" onmouseout="this.style.background='#cccccc'" onclick="parent.location.href='http://search.microsoft.com';">
Search</DIV>
<DIV STYLE="position:relative; top:0; left:0; background:#cccccc; border:1px solid black; border-top: 1px solid white; border-left:1px solid white; height:20px; color:black; font-family:verdana; font-weight:bold; padding:2px; padding-left:10px; font-size:8pt; cursor:hand" onmouseover="this.style.background='#ffffff'" onmouseout="this.style.background='#cccccc'" onclick="parent.location.href='http://www.microsoft.com/ie';">
Intenet Explorer</DIV>
<DIV STYLE="position:relative; top:0; left:0; background:#cccccc; border:1px solid black; border-top: 1px solid white; border-left:1px solid white; height:20px; color:black; font-family:verdana; font-weight:bold; padding:2px; padding-left:10px; font-size:8pt; cursor:hand" onmouseover="this.style.background='#ffffff'" onmouseout="this.style.background='#cccccc'" onclick="parent.location.href='http://www.microsoft.com/info/cpyright.htm';">
?2001 Microsoft Corporation</DIV>
</DIV>
</body>
</html>
Only one thing is very import.if you want to display your page in mainFrame.you need change the onclick method.the syntax is :
parent.parent.mainFrame.location.href='http://www.microsoft.com';A busy day,do many things
Today is a busy day,for review some requirement,I must read a large size of document,and ask some related guys to clairy every thing.
Although it's a busy day,but I really feel good,because I know a lot of what I don't know things before.Greate!!!
Wednesday, April 9, 2008
How to for "No moudle named zope.interface"
"No moudle named zope.interface."
That means the "twisted.internet" package depends on the zope.interface page.So,how should we do?especially in windows paltform.The solution is download the zope.inteface package and install it.
you can download it from:http://www.zope.org/Products/ZopeInterface/3.1.0b1/zope.interface-3.1.0b1.win32-py2.4.exe
As you can see,for now,it's only include the version for python 2.4 .for python2.5 you must install from source code.
Thursday, March 20, 2008
I'd better to write a several of tutorial for basic of Python.
Monday, March 17, 2008
Getting start with Django
Create Project and Config data base.
1) create project
For one thing,of course,we need download the Django and setup it.I decide to grap the last version of Django,so I setup the Subverion client,and then check out the last Django trunk.
svn co http://code.djangoproject.com/svn/django/trunk djtrunk
And then put my djtrunk path to PYTHONPATH environment variables.
finally,we place our djtrunk/django/bin on our system path.because this directary includes management utilities such as django-admin.py.
For now,everything is OK,we'll create our first application in Django environment.It's easy to create a project.Open your django project directory and just type following command.
django-admin.py startproject sparrow
Note:sparrow is our project's name,you can change as everything you like.
After run this command,Django will create a sparrow directory.and this directory include four files:
- __init__.py: A file required for Python treat the directory as a package (i.e., a group of modules)
- manage.py: A command-line utility that lets you interact with this Django project in various ways
- settings.py: Settings/configuration for this Django project
- urls.py: The URL declarations for this Django project; a “table of contents” of your Django-powered site
python manage.py runserver
you will see some log information,and the server is run on port 8000(default).Then open your browser and open folling url:http://127.0.0.1"8000.You will see a congratulation page with some default legend.Django includes a built-in, lightweight Web server you can use while developing your site. We’ve included this server so you can develop your site rapidly, without having to deal with configuring your production Web server (e.g., Apache) until you’re ready for production.
2) Config data base for Django project.
As of now,Django support three type DataBase:
- PostgreSQL (http://www.postgresql.org/)
- SQLite 3 (http://www.sqlite.org/)
- MySQL (http://www.mysql.com/)
First of all,we'll download the PostgreSQL database engine and download the python postgresql package.
http://www.djangoproject.com/r/python-pgsql/. Take note of whether you’re using version 1 or 2; you’ll need this information later.
Windows user can download from http://www.djangoproject.com/r/python-pgsql/windows/.
Now,we'll config the database in Django.please open the file settings.py in sparrow directoary.
and find the section DATABASE_ENGINE,and change it like this;
DATABASE_ENGINE = 'postgresql_psycopg2' # 'postgresql_psycopg2', 'postgresql', 'mysql', 'sqlite3' or 'oracle'.
DATABASE_NAME = 'test2' # Or path to database file if using sqlite3.
DATABASE_USER = 'postgres' # Not used with sqlite3.
DATABASE_PASSWORD = '123456' # Not used with sqlite3.
DATABASE_HOST = '127.0.0.1' # Set to empty string for localhost. Not used with sqlite3.
DATABASE_PORT = '5432' # Set to empty string for default. Not used with sqlite3.
Congratulation,we have our first Django application and config it with postgreSQL.It's cool.
In next chapter we'll discuss how to create a Domain model,it'll map to real database table.
Monday, March 10, 2008
How to add and remove apache server as a WinNT service
open the directory of apache installed.then type following command.
>>httpd -k install
then apache server be installed as a windows service.And we also can saw it in apache monitor,it's easily to maintain now.In the other hand ,if we need remove it from windows service.type following command
>>httpd -k uninstall.
and start apache with command
>>httpd -k start.
and stop apache with command
>>httpd -k stop.
and restart apache with command
>>httpd -k restart.